Angular and Bootstrap are very useful tools when developing front ends. You can build single page applications with powerful features of Angular and make them look pretty by using Bootstrap classes and components. They both have new versions now, coincidentally each has version 4. However, Bootstrap 4 is currently in beta stage.
In this blog post I will explain how to import Bootstrap 4 Sass module in an Angular 4 application as SCSS.
UPDATE - December 5, 2017: Angular recently launched version 5.0.0 and the method in this post also apply to Angular 5, too.
Create a new Angular 4 app to use SCSS
By default Angular 4 applications use css as style files. However, this can be modified. To achieve this, we only include a --style
attribute in our call while creating our application using Angular CLI.
ng new my-new-app --style=scss
Here “my-new-app” is the name of our application. You can substitute it with a name that you choose for your application.
Install Ng-Bootstrap Module
Starting from Angular 2, there is an integrated module for Bootstrap 4 javascript components: NgBootstrap. Now, let’s include it in our application. We should first go to our app folder and then install @ng-bootstrap module using npm.
cd my-new-app
npm install --save @ng-bootstrap/ng-bootstrap
Now, let’s integrate this module into our app.module.ts file. Please see how we imported NgbModule into typescript file and imported in AppModule class in NgModule decorator.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import {NgbModule} from '@ng-bootstrap/ng-bootstrap';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
NgbModule.forRoot()
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Install Bootstrap Sass and Import
Now, we need to install Bootstrap 4 and import its main scss file into our application. Ng-bootstrap only installs Typescript components. We need to install Bootstrap without including Javascript files.
npm install --save bootstrap@4.0.0-beta.2
Then we need to import Bootstrap Sass into our application. In styles.scss add this line:
@import "../node_modules/bootstrap/scss/bootstrap.scss";
Customize Bootstrap Variables
I prefer making a separate file for putting all bootstrap customizations such as link colors. Let us create “styles” folder in “src/assets” and create a file named “_bootstrap_customizations.scss”
mkdir src/assets/styles
Then change link color as red in all our application using bootstrap’s $link-color variable.
$link-color: red !default;
To make this take effect globally in our application, we import this file in our styles.scss before bootstrap. Now our styles.cscc becomes:
@import "./assets/styles/bootstrap_customizations.scss";
@import "../node_modules/bootstrap/scss/bootstrap.scss";
Test
To test all these let us create a container and card in our app.component.html and put some text and link there.
Change app.component.html by deleting all and inserting:
<div class="container">
<div class="card">
<div class="card-body">
<h1>Hello World!</h1>
<p>
<a href="#">Link to Mars</a>
</p>
</div>
</div>
</div>
Run the application using angluar-cli.
ng serve
Open your browser and navigate to “localhost:4200” and the result should be similar to this:
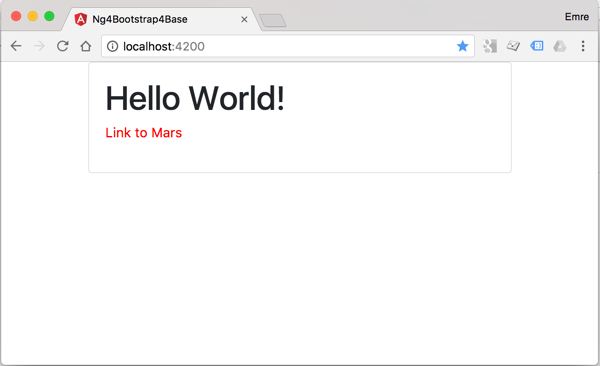
Conclusion
As can be seen, it is very straightforward to include Bootstrap 4 Sass module into Angular 2/4/5 applications.
You can find a sample application for an implementation of this integration our Github repository.
Thanks for reading!